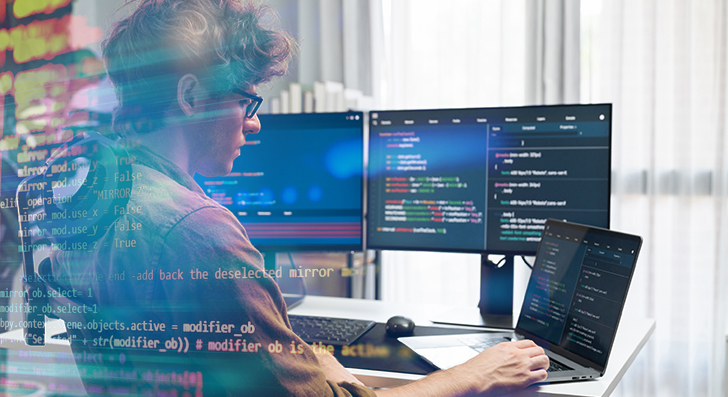
Scalability means your application can manage development—extra people, far more information, and much more website traffic—devoid of breaking. Like a developer, creating with scalability in mind will save time and anxiety later. Below’s a clear and sensible guide that may help you commence by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on later—it ought to be portion of your system from the beginning. Quite a few applications fall short when they improve quick because the initial design can’t take care of the additional load. Like a developer, you might want to Believe early regarding how your system will behave under pressure.
Start by planning your architecture to be versatile. Prevent monolithic codebases exactly where almost everything is tightly related. Rather, use modular design and style or microservices. These patterns split your application into smaller, impartial areas. Each module or services can scale on its own devoid of influencing The full procedure.
Also, think about your database from day one particular. Will it have to have to handle a million consumers or maybe a hundred? Choose the correct variety—relational or NoSQL—based upon how your details will grow. Strategy for sharding, indexing, and backups early, even if you don’t want them nevertheless.
Another essential level is in order to avoid hardcoding assumptions. Don’t publish code that only will work underneath present-day disorders. Think about what would happen In the event your consumer base doubled tomorrow. Would your app crash? Would the database slow down?
Use style patterns that assistance scaling, like message queues or event-pushed programs. These support your app take care of far more requests with no receiving overloaded.
If you Construct with scalability in mind, you are not just getting ready for achievement—you are decreasing long term headaches. A nicely-planned system is easier to keep up, adapt, and develop. It’s better to get ready early than to rebuild later on.
Use the correct Database
Choosing the suitable databases is really a essential Portion of developing scalable purposes. Not all databases are designed precisely the same, and using the Completely wrong you can slow you down or even trigger failures as your application grows.
Start off by comprehending your details. Could it be extremely structured, like rows inside of a table? If yes, a relational databases like PostgreSQL or MySQL is an effective match. These are solid with relationships, transactions, and regularity. They also guidance scaling methods like browse replicas, indexing, and partitioning to deal with extra targeted traffic and info.
If your knowledge is a lot more versatile—like person activity logs, merchandise catalogs, or documents—take into account a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at handling massive volumes of unstructured or semi-structured data and may scale horizontally far more very easily.
Also, consider your go through and produce patterns. Have you been accomplishing numerous reads with fewer writes? Use caching and read replicas. Will you be managing a hefty publish load? Take a look at databases that may take care of significant write throughput, or maybe party-based info storage programs like Apache Kafka (for non permanent data streams).
It’s also intelligent to Feel forward. You might not will need advanced scaling characteristics now, but picking a databases that supports them usually means you received’t need to switch later.
Use indexing to speed up queries. Steer clear of needless joins. Normalize or denormalize your info dependant upon your obtain styles. And always monitor database performance as you grow.
To put it briefly, the proper database is determined by your application’s framework, pace demands, And exactly how you be expecting it to improve. Acquire time to choose properly—it’ll preserve plenty of difficulty afterwards.
Enhance Code and Queries
Quickly code is key to scalability. As your app grows, every compact hold off adds up. Badly written code or unoptimized queries can decelerate effectiveness and overload your system. That’s why it’s imperative that you Establish successful logic from the start.
Begin by crafting cleanse, basic code. Stay away from repeating logic and remove just about anything unneeded. Don’t choose the most sophisticated Answer if a straightforward one particular functions. Keep the features brief, concentrated, and simple to check. Use profiling instruments to locate bottlenecks—places where by your code can take as well extensive to run or uses an excessive amount memory.
Up coming, look at your database queries. These often sluggish things down in excess of the code by itself. Make sure Every single query only asks for the information you truly want. Stay clear of Pick *, which fetches all the things, and as an alternative find certain fields. Use indexes to speed up lookups. And prevent doing too many joins, Primarily throughout big tables.
When you notice precisely the same details getting asked for many times, use caching. Retail store the results briefly working with tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database operations any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and will make your app much more productive.
Make sure to exam with large datasets. Code and queries that function fantastic with one hundred data could possibly crash when they have to handle 1 million.
Briefly, scalable applications are rapid applications. Keep the code limited, your queries lean, and use caching when desired. These steps help your application stay smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with far more end users and a lot more website traffic. If every little thing goes by just one server, it can immediately become a bottleneck. That’s the place load balancing and caching are available in. These two instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of one particular server undertaking each of the function, the load balancer routes customers to different servers according to availability. This means no one server will get overloaded. If a person server goes down, the load balancer can send out traffic to the others. Applications like Nginx, HAProxy, or cloud-dependent answers from AWS and Google Cloud make this easy to arrange.
Caching is about storing knowledge temporarily so it might be reused promptly. website When buyers request exactly the same information and facts yet again—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may serve it within the cache.
There are 2 common different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers data in memory for rapidly access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents near the user.
Caching lessens database load, improves pace, and makes your application far more economical.
Use caching for things that don’t improve frequently. And generally make certain your cache is up-to-date when data does adjust.
In short, load balancing and caching are straightforward but impressive tools. Collectively, they assist your application cope with much more end users, continue to be quick, and Get well from complications. If you plan to expand, you require both.
Use Cloud and Container Equipment
To develop scalable purposes, you require tools that let your app increase quickly. That’s where cloud platforms and containers come in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to lease servers and expert services as you'll need them. You don’t must get components or guess long run ability. When targeted visitors improves, you can add much more sources with just a few clicks or immediately utilizing auto-scaling. When visitors drops, you are able to scale down to save money.
These platforms also offer you companies like managed databases, storage, load balancing, and safety equipment. You'll be able to give attention to developing your app instead of running infrastructure.
Containers are A different critical Resource. A container deals your app and everything it really should operate—code, libraries, options—into 1 unit. This can make it uncomplicated to move your app concerning environments, from the laptop computer to the cloud, without the need of surprises. Docker is the preferred Device for this.
When your application employs numerous containers, applications like Kubernetes make it easier to deal with them. Kubernetes handles deployment, scaling, and recovery. If 1 part of your respective app crashes, it restarts it quickly.
Containers also help it become simple to different areas of your app into expert services. You'll be able to update or scale parts independently, that's great for effectiveness and reliability.
To put it briefly, making use of cloud and container applications signifies you can scale speedy, deploy simply, and Get better quickly when challenges occur. In order for you your app to increase without limitations, get started making use of these instruments early. They save time, lessen hazard, and enable you to continue to be focused on creating, not correcting.
Check All the things
In the event you don’t keep an eye on your software, you won’t know when items go Erroneous. Checking helps you see how your application is undertaking, location problems early, and make greater conclusions as your application grows. It’s a key Portion of constructing scalable units.
Start by tracking primary metrics like CPU use, memory, disk space, and response time. These let you know how your servers and providers are executing. Applications like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—keep an eye on your application way too. Control how much time it will require for buyers to load internet pages, how frequently faults materialize, and where they occur. Logging resources like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly can assist you see what’s taking place inside your code.
Setup alerts for essential issues. As an example, Should your response time goes above a Restrict or simply a company goes down, you'll want to get notified promptly. This can help you correct troubles quickly, frequently prior to users even see.
Checking is additionally helpful once you make changes. Should you deploy a whole new characteristic and see a spike in glitches or slowdowns, it is possible to roll it back before it will cause serious destruction.
As your app grows, visitors and details enhance. Without having checking, you’ll overlook signs of issues right up until it’s as well late. But with the ideal equipment in place, you stay on top of things.
In short, checking assists you keep the app trusted and scalable. It’s not nearly recognizing failures—it’s about knowing your procedure and ensuring it really works effectively, even stressed.
Last Views
Scalability isn’t just for massive businesses. Even smaller apps need to have a strong foundation. By coming up with very carefully, optimizing correctly, and using the proper applications, you may Develop applications that mature easily without breaking under pressure. Start out small, Feel significant, and Develop sensible.